Threads are a fundamental concept in computer science and operating systems. In simple terms, a thread refers to an independent sequence of execution within a program. It is a lightweight unit of a process, capable of performing tasks concurrently with other threads.
Threads are executed within a process and share the same memory space, allowing them to access and modify data in the process. Multiple threads within a process can work on different parts of a task simultaneously, which can lead to increased efficiency and responsiveness in applications.
Here are a few key points about threads:
- Threads are sometimes referred to as “lightweight processes” because they are smaller and faster to create than traditional processes.
- Threads within a process share the same resources, such as memory, file handles, and open network connections, reducing the need for inter-process communication mechanisms.
- Each thread has its own program counter, stack, and set of registers, which enable independent execution.
- Threads can communicate and synchronize with each other through mechanisms like shared memory, locks, semaphores, and condition variables.
- Threads can be used for various purposes, such as handling user input, performing background tasks, parallelizing computations, and managing concurrent I/O operations.
- Multithreading is the practice of using multiple threads within a program to achieve parallelism and improve overall performance.
It’s worth noting that threads can introduce complexities related to concurrency and synchronization. Proper management and synchronization of shared resources are crucial to avoid issues like race conditions, deadlocks, and data corruption.
Threads are widely used in modern programming languages and frameworks to develop efficient and responsive software applications.
Features
Concurrency
Concurrency in threads refers to the ability of multiple threads within a program to execute independently and make progress simultaneously. It allows for the overlapping or interleaved execution of tasks, leading to increased efficiency and responsiveness in software applications.
Concurrency is essential in scenarios where multiple tasks need to be performed concurrently, such as handling user input, performing background operations, or managing concurrent I/O operations. By utilizing threads, developers can design applications that make progress on multiple tasks simultaneously, improving overall performance.
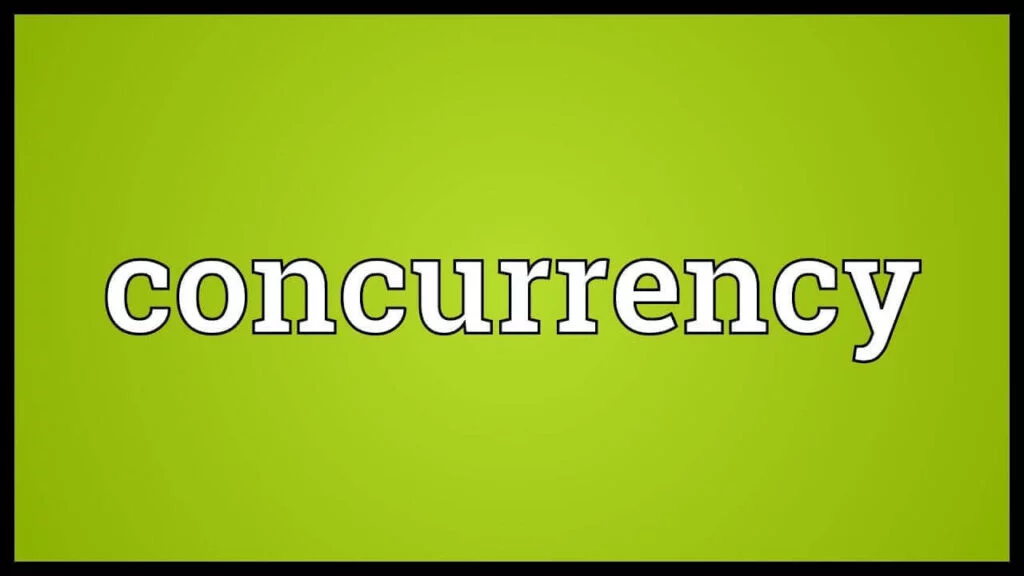
There are several key aspects to consider when discussing concurrency in threads:
- Independent Execution: Each thread within a program has its own sequence of instructions to execute independently. These threads can perform different operations or work on different parts of a task simultaneously. For example, in a web server application, multiple threads can handle incoming client requests concurrently.
- Overlapping Execution: Concurrency allows threads to overlap their execution in time. While one thread is waiting for an I/O operation to complete, another thread can execute and continue making progress on a different task. This overlapping of execution reduces idle time and maximizes the utilization of system resources.
- Context Switching: Context switching is the mechanism by which the operating system switches the execution context from one thread to another. It allows threads to take turns executing on a single processor core, giving the illusion of simultaneous execution. Context switching typically occurs when a thread blocks or voluntarily yields the CPU.
- Synchronization: Since threads share the same memory space, they may access and modify shared data concurrently. Proper synchronization mechanisms, such as locks, semaphores, or atomic operations, are crucial to prevent race conditions and ensure thread-safe access to shared resources. Synchronization mechanisms provide coordination and enforce order among threads, ensuring correct and predictable behavior.
- Resource Management: Concurrency requires careful management of shared resources, such as memory, file handles, or network connections. Thread-safe data structures and synchronization techniques must be employed to prevent conflicts and data corruption. Additionally, resource pooling techniques can be used to efficiently manage limited resources among multiple threads.
- Communication: Threads often need to communicate with each other to exchange data or coordinate their actions. Communication mechanisms, such as message queues, pipes, or shared memory, enable threads to pass messages or share information efficiently. Effective communication between threads is essential for synchronization and collaboration in concurrent applications.
- Scalability: Concurrency plays a crucial role in achieving scalability in applications. By utilizing multiple threads, developers can take advantage of the available computing resources, such as multicore processors or distributed systems. Dividing tasks into smaller units of work that can be executed concurrently allows for better utilization of system resources and improved performance.
- Deadlocks and Race Conditions: Concurrency introduces challenges like deadlocks and race conditions. Deadlocks occur when two or more threads are waiting for each other to release resources, resulting in a stalemate. Race conditions occur when multiple threads access shared resources concurrently, leading to unpredictable behavior or data corruption. Proper synchronization techniques and careful programming practices are necessary to mitigate these issues.
In conclusion, concurrency in threads allows for the simultaneous execution of multiple tasks within a program. It improves efficiency, responsiveness, and scalability in software applications. However, it also introduces challenges related to synchronization, resource management, and potential issues like deadlocks and race conditions. By understanding and appropriately addressing these challenges, developers can harness the power of concurrency to create robust and efficient concurrent software systems.
Lightweight
In the context of threads, the term “lightweight” refers to their efficiency and low overhead compared to full processes. Lightweight threads, also known as “user-level threads” or “green threads,” provide a way to achieve concurrent execution within a single process without the need for operating system involvement or management. Here’s a closer look at what makes threads lightweight:
- Creation and Termination: Creating a thread is faster and requires fewer system resources compared to creating a new process. Thread creation typically involves allocating a small amount of memory for the thread’s stack and initializing its execution context. Similarly, terminating a thread is also quicker as it involves cleaning up its resources without the need to tear down the entire process.
- Switching and Scheduling: Context switching between threads is faster compared to process context switches. When a thread yields the CPU or encounters a blocking operation, the operating system’s thread scheduler can quickly switch to another ready thread within the same process. This lightweight switching enables efficient multitasking and allows threads to make progress without the overhead of full process context switches.
- Resource Sharing: Threads within a process share the same memory space, file handles, network connections, and other system resources. Since threads can directly access shared data and resources without the need for complex inter-process communication mechanisms, the overhead of resource sharing is reduced. This streamlined access to shared resources enhances efficiency and avoids the need for costly data transfers between processes.
- Communication and Synchronization: Lightweight threads can communicate and synchronize with each other using mechanisms like shared memory, locks, semaphores, or condition variables. These mechanisms are implemented at the user level and do not involve the operating system’s intervention, making them more efficient and lightweight compared to inter-process communication mechanisms used by separate processes.
- Scalability: Lightweight threads are designed to scale efficiently, allowing the application to take advantage of modern multicore processors and distributed computing environments. By dividing a task into multiple lightweight threads, the application can leverage the available computing resources more effectively, achieving parallelism and improved performance.
- Flexibility and Portability: Lightweight threads are typically implemented at the application level or within a runtime library, making them independent of the underlying operating system. This provides greater flexibility and portability, as applications can be developed and run on different platforms without significant modifications. The application itself manages the scheduling and execution of lightweight threads, tailoring it to its specific requirements.
- Lower Memory Footprint: Lightweight threads generally have a smaller memory footprint compared to processes. Since threads within a process share the same memory space, they do not require the duplication of code or data segments, resulting in reduced memory usage. This can be particularly advantageous when a large number of concurrent tasks need to be executed.
It’s important to note that while lightweight threads offer benefits in terms of efficiency and flexibility, they also require careful management and synchronization. Developers need to ensure proper synchronization mechanisms and avoid issues like race conditions or deadlocks when multiple threads access shared resources.
In summary, lightweight threads provide a more efficient and lightweight approach to achieving concurrency within a single process. They offer faster creation and termination, lightweight context switching, streamlined resource sharing, efficient communication and synchronization, scalability, flexibility, and reduced memory footprint. By leveraging lightweight threads, developers can create concurrent applications that maximize efficiency and responsiveness while minimizing resource overhead.
Communication and Synchronization
Communication and synchronization are fundamental concepts in concurrent programming, enabling threads or processes to interact, exchange data, and coordinate their actions. They play a crucial role in managing shared resources, avoiding conflicts, and ensuring the correct and orderly execution of concurrent tasks. Here’s a closer look at communication and synchronization:
- Communication: Communication refers to the mechanism by which threads or processes share information, exchange messages, or transfer data. It allows concurrent entities to cooperate, coordinate their activities, and work together towards a common goal. Communication can take different forms:
- Shared Memory: Threads or processes can communicate by accessing shared variables or data structures residing in a shared memory region. They can read from or write to these shared locations to exchange information. Care must be taken to ensure proper synchronization to avoid data races or inconsistencies.
- Message Passing: In message passing communication, threads or processes explicitly send and receive messages or signals to communicate. Messages can contain data, instructions, or requests. The communication can be one-to-one or involve multiple entities using point-to-point or multicast communication.
- Pipes and Channels: Pipes and channels provide a means of communication between processes or threads through a unidirectional or bidirectional flow of data. They enable data transfer or synchronization by acting as conduits for communication.
- Sockets and Networking: Communication can also occur between processes or threads over networks using sockets. This enables distributed communication across different machines or processes running on different hosts.
- Synchronization: Synchronization is the coordination of concurrent threads or processes to ensure orderly and consistent execution. It involves enforcing order, preventing conflicts, and managing shared resources. Key synchronization mechanisms include:
- Locks and Mutexes: Locks, also known as mutexes (mutual exclusion), are used to provide exclusive access to shared resources. Threads or processes acquire locks before accessing the resource, ensuring that only one entity can access it at a time. This prevents data races and maintains consistency.
- Semaphores: Semaphores are synchronization objects that control access to a shared resource based on a counter. They can be used to limit the number of threads or processes accessing a resource simultaneously or to signal the availability of resources.
- Condition Variables: Condition variables allow threads to wait for a certain condition to become true before proceeding. They are often used in conjunction with locks or mutexes to manage the flow of execution and synchronize threads.
- Barriers: Barriers allow a group of threads or processes to wait until all participants have reached a certain point in their execution before continuing. Barriers are useful when multiple threads need to synchronize and coordinate their activities.
- Atomic Operations: Atomic operations guarantee that a specific operation is performed as a single, indivisible unit, without interference from other threads or processes. They are typically used to ensure safe access to shared variables or perform simple synchronization tasks.
Communication and synchronization often go hand in hand. They are intertwined concepts, as proper synchronization is essential for safe and reliable communication between concurrent threads or processes. By utilizing effective communication and synchronization techniques, developers can avoid issues like data races, deadlocks, and inconsistent state, ensuring the correct execution and reliability of concurrent applications.
Scheduling
Scalability is a crucial concept in computer systems and software design, referring to the ability of a system to handle increasing workloads and growing demands while maintaining or improving its performance, responsiveness, and efficiency. Scalability ensures that a system can adapt and expand to meet the requirements of a growing user base or increasing data volume.
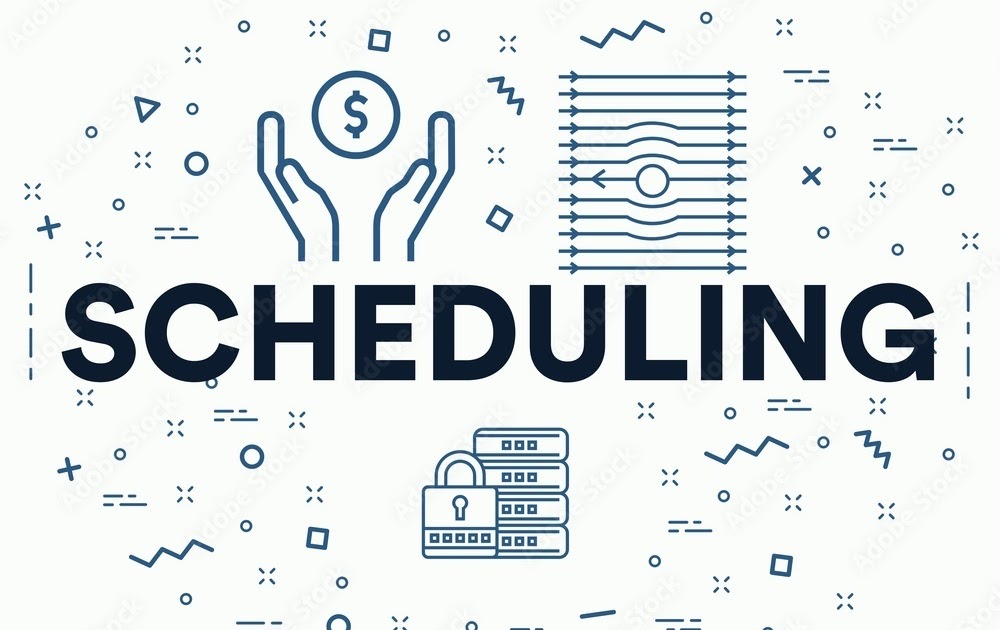
Here’s a closer look at scalability:
- Horizontal Scalability: Horizontal scalability, also known as scale-out, involves adding more machines, servers, or nodes to a system to handle increasing workloads. By distributing the load across multiple machines, horizontal scalability allows for improved performance and increased capacity. This approach is often used in distributed systems, cloud computing, or web applications, where additional servers can be added to the system as needed.
- Vertical Scalability: Vertical scalability, also known as scale-up, involves increasing the resources (such as CPU, memory, or storage) of a single machine to handle growing workloads. Vertical scalability typically involves upgrading or replacing hardware components to enhance the system’s capacity. This approach is useful when a system reaches the limits of a single machine’s capabilities or when the cost of adding additional machines is not feasible.
- Load Balancing: Load balancing is a technique used to distribute workloads evenly across multiple machines or resources to achieve optimal utilization and performance. Load balancers can intelligently route incoming requests or tasks to available resources, ensuring that the workload is evenly distributed and preventing any single resource from becoming overwhelmed. Load balancing contributes to scalability by efficiently utilizing resources and avoiding bottlenecks.
- Partitioning and Sharding: Partitioning, also known as sharding, involves dividing a large dataset or workload into smaller, manageable parts and distributing them across multiple resources. Each partition or shard can be handled independently, allowing for parallel processing and improved performance. Partitioning is commonly used in distributed databases or data-intensive applications to achieve scalability and handle large volumes of data.
- Elasticity: Elasticity refers to the ability of a system to automatically and dynamically scale resources up or down based on demand. With elastic systems, resources can be added or removed dynamically as workload requirements fluctuate. This approach ensures that the system can efficiently allocate resources when demand is high and release them when demand decreases, optimizing resource utilization and cost-effectiveness.
- Performance and Throughput: Scalability aims to maintain or improve system performance and throughput as the workload or user base grows. A scalable system can handle increased requests or transactions without a significant degradation in response time or throughput. By effectively distributing workloads, utilizing resources efficiently, and minimizing bottlenecks, scalability helps ensure that system performance remains consistent even under high loads.
- Concurrency and Parallelism: Scalability often involves leveraging concurrency and parallelism to process tasks simultaneously and take advantage of available computing resources. By dividing tasks into smaller units that can be executed concurrently or in parallel, a scalable system can make efficient use of multiple processors, cores, or machines, thereby achieving improved performance and scalability.
- Redundancy and Fault Tolerance: Scalable systems often incorporate redundancy and fault tolerance mechanisms to ensure high availability and reliability. By replicating critical components or data across multiple machines or data centers, a scalable system can continue functioning even if individual resources fail. Redundancy helps prevent single points of failure and enhances the system’s ability to handle increasing workloads without disruptions.
Scalability is a crucial consideration in the design and implementation of systems, particularly for those expected to grow or handle varying workloads. By employing strategies such as horizontal or vertical scaling, load balancing, partitioning, elasticity, and fault tolerance, developers can create systems that can grow and adapt to meet the evolving needs of users and handle increasing demands while maintaining performance and reliability.
Parallelism
Parallelism is a concept in computer science that involves breaking down a task into smaller subtasks and executing them simultaneously, often with the goal of improving efficiency and reducing execution time. It allows for the concurrent execution of multiple tasks to leverage the available computing resources, such as multiple processors, cores, or machines. Parallelism is crucial in modern computing to exploit the power of multicore processors and high-performance computing systems.
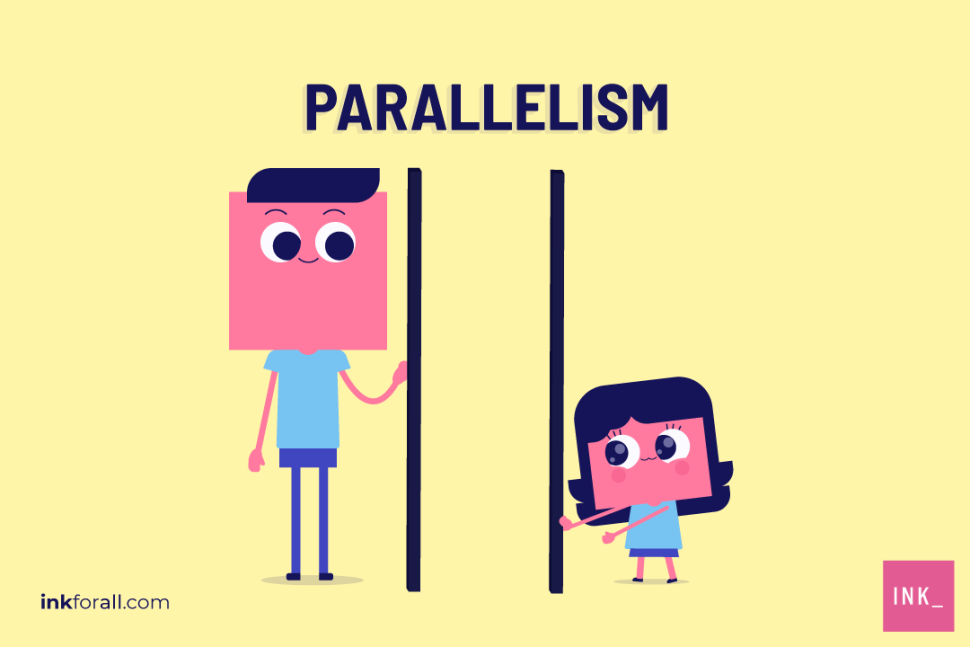
Here’s a closer look at parallelism:
- Task Decomposition: Parallelism starts with task decomposition, where a large task is divided into smaller, independent subtasks that can be executed concurrently. This decomposition can be achieved through various techniques, such as dividing a dataset into smaller parts, breaking down a computation into parallelizable operations, or partitioning work based on different parameters.
- Simultaneous Execution: Parallelism enables the simultaneous execution of multiple subtasks on different processing units. These units can be multiple cores within a single processor, multiple processors within a system, or even distributed systems with multiple machines. Each processing unit works on a different subtask, allowing for faster execution and improved performance.
- Speedup and Efficiency: The main advantage of parallelism is the potential for speedup, where the total execution time of a task is reduced by executing subtasks in parallel. Speedup is achieved when the total time taken to complete the task with parallel execution is less than the time taken for sequential execution. Efficiency measures how effectively the parallel execution utilizes the available resources, minimizing overhead and maximizing throughput.
- Types of Parallelism: Parallelism can be classified into different types based on the level at which tasks are divided and executed:
- Data Parallelism: In data parallelism, the same operation is performed on different subsets of data in parallel. It involves dividing the data into smaller chunks and executing the same operation on each chunk simultaneously. This type of parallelism is often used in applications that process large datasets, such as image or video processing.
- Task Parallelism: Task parallelism involves executing different tasks or operations concurrently. Each task works on a separate portion of the overall problem, and they can be executed independently or interdependently. Task parallelism is suitable for applications with diverse and independent operations, such as parallelizing independent computations or performing concurrent I/O operations.
- Instruction-level Parallelism: Instruction-level parallelism focuses on parallelizing the execution of instructions within a single task or program. This type of parallelism exploits dependencies and independent instructions to execute them simultaneously, optimizing the use of CPU pipelines or superscalar architectures.
- Parallel Programming Models: Parallelism in software is achieved through parallel programming models and libraries that provide abstractions and tools for managing parallel execution. Some popular parallel programming models include:
- Shared-memory programming models (e.g., OpenMP): These models utilize shared memory to allow multiple threads or processes to access and modify shared data. They use constructs like parallel loops or directives to express parallelism and handle synchronization.
- Message-passing programming models (e.g., MPI): Message-passing models facilitate communication between different tasks or processes by sending and receiving messages. They are commonly used in distributed memory systems, where tasks run on separate machines and communicate through explicit message passing.
- Dataflow programming models (e.g., TensorFlow): Dataflow models express computation as a directed graph, where nodes represent operations, and edges represent data dependencies. This model allows for parallel execution of operations as soon as their input data becomes available.
- Granularity: Granularity refers to the size of the subtasks in parallel execution. Fine-grained parallelism involves small subtasks, allowing for more parallelism but potentially incurring higher overhead due to communication and synchronization. Coarse-grained parallelism involves larger subtasks, reducing overhead but potentially limiting the level of parallelism. The choice of granularity depends on factors such as the nature of the task, available resources, and communication overhead.
Parallelism is vital for achieving high performance and efficient utilization of computing resources. It enables faster execution, increased throughput, and the ability to handle larger and more complex tasks. By effectively decomposing tasks, utilizing appropriate parallel programming models, and considering factors like data dependencies and granularity, developers can harness the power of parallelism to create high-performance computing applications.
Deadlocks and Race Conditions
Deadlocks and race conditions are two common issues that can occur in concurrent programming when multiple threads or processes interact with shared resources. They can lead to unexpected and undesirable behavior, impacting the correctness, reliability, and performance of the system. Here’s a closer look at deadlocks and race conditions:
- Deadlocks: A deadlock occurs when two or more threads or processes are blocked indefinitely, waiting for each other to release resources that they hold. Deadlocks typically occur when the following four conditions are met simultaneously:
- Mutual Exclusion: The resources involved are non-sharable and can be held by only one thread or process at a time.Hold and Wait: A thread or process holds at least one resource while waiting to acquire additional resources.No Preemption: Resources cannot be forcibly taken away from a thread or process.Circular Wait: A circular chain of threads or processes exists, with each waiting for a resource held by the next entity in the chain.
- Race Conditions: A race condition occurs when the behavior or outcome of a program depends on the relative timing or interleaving of events in multiple threads or processes. It arises when multiple threads access shared resources concurrently, and at least one thread performs a non-atomic operation (an operation that is not indivisible). Race conditions can lead to unpredictable and inconsistent results.Common types of race conditions include:
- Read-Modify-Write: When multiple threads read, modify, and write a shared variable simultaneously, the final value of the variable may depend on the interleaving of operations, leading to inconsistent results.Check-Then-Act: In situations where a thread checks the state of a shared resource and then performs an action based on that state, other threads may change the resource’s state between the check and the action, causing unexpected behavior.Order of Operations: When multiple threads perform a series of operations on shared resources without proper synchronization, the order of operations may differ between threads, leading to inconsistent or incorrect results.
- Prevention and Mitigation: To prevent and mitigate deadlocks and race conditions, the following strategies can be employed:
- Synchronization: Proper synchronization using locks, mutexes, semaphores, or other mechanisms can ensure exclusive access to shared resources and prevent race conditions. Synchronization mechanisms enforce order and avoid conflicts between threads.
- Avoidance of Circular Waits: Designing resource allocation algorithms and strategies to avoid circular wait conditions can prevent deadlocks. This may involve imposing a partial ordering on resources or using techniques like resource request ordering.
- Deadlock Detection and Recovery: Implementing deadlock detection algorithms can identify and resolve deadlocks. Techniques like resource preemption, where a higher-priority process can forcibly acquire resources from a lower-priority process, can be used for deadlock recovery.
- Proper Resource Management: Careful resource management practices, such as limiting resource allocation, releasing resources promptly when no longer needed, and avoiding unnecessary resource holding, can reduce the likelihood of deadlocks and increase system efficiency.
- Testing and Debugging: Thorough testing, including stress testing with high concurrency, can help uncover and address potential deadlocks and race conditions. Debugging techniques like logging, tracing, and analyzing thread interactions can assist in identifying and resolving these issues.
Deadlocks and race conditions are important considerations in concurrent programming. By understanding their causes, employing appropriate synchronization techniques, and following best practices for resource management, developers can minimize the occurrence of these issues, ensuring the correctness, reliability, and performance of concurrent software systems.